Step 5: Watch a transaction status
To fetch the execution status of a transfer, the /status endpoint can be queried. View the full API reference for the
/status
endpoint here.
The next step in our transaction flow is a status check. You can check the status of a transaction by querying the /status
endpoint.
Checking a transaction's status is important because it has two (2) essential functions:
- It let's you know the current state of your transaction.
- Most importantly, it also reports the
txHash
of your transaction to Swing, which enables Swing to track your transaction on the blockchain.
It's important to note that if the /status
endpoint is not queried at least once after a transaction is sent, the transaction will not appear in the Transactions
section of your platform's dashboard. Once the transaction's status has been queried, it will be displayed on your dashboard:
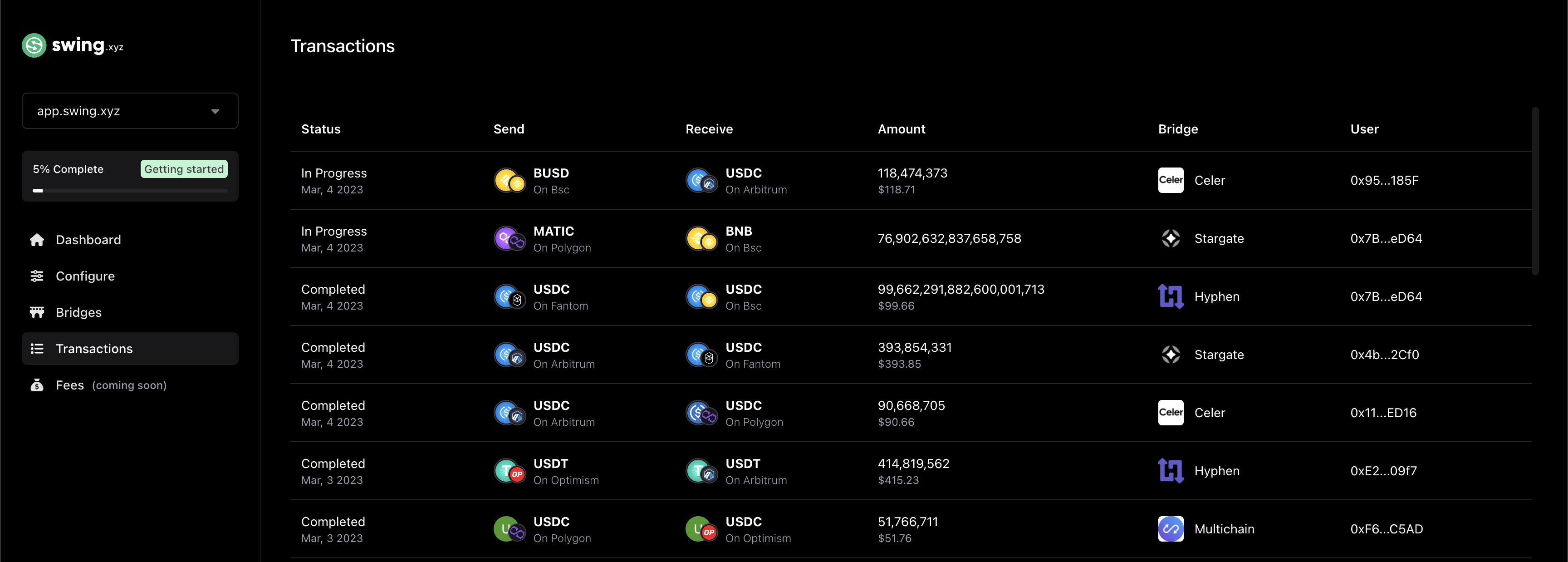
Making a Request
GET: https://swap.prod.swing.xyz/v0/transfer/status
Query Parameters:
Key | Example | Description |
---|---|---|
id | 239750 | Transaction ID from /send response |
txHash | 0x3b2a04e2d16489bcbbb10960a248..... | The transaction hash identifier. |
projectId | replug | Your project's ID |
Sample Request
const result = await axios.get(
'https://swap.prod.swing.xyz/v0/transfer/status',
{
params: {
id: 239750,
txHash: '0x3b2a04e2d16489bcbbb10960a248bcbbb1090000..........',
projectId: 'replug',
},
},
);
Response
{
"status": "Completed",
"refundReason": "",
"errorReason": null,
"needClaim": false,
"bridge": "dodo",
"txId": "s1712147672836",
"txStartedTimestamp": 1712147713,
"txCompletedTimestamp": 1712147713,
"fromUserAddress": "0x018c15da1239b84b08283799b89045cd476bbbbb",
"toUserAddress": "0x018c15da1239b84b08283799b89045cd476bbbbb",
"fromTokenAddress": "0x3c499c542cef5e3811e1192ce70d8cc03d5c3359",
"fromAmount": "400000",
"fromAmountUsdValue": "0.4",
"fromChainId": 137,
"fromChainSlug": "polygon",
"fromChainTxHash": "0x0554846ce55a79b5b635230263a7252c6b7749d1c32a845185e5f35d1aeb150b",
"toTokenAddress": "0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee",
"toAmount": "443119108223201734",
"toAmountUsdValue": "0.402",
"toChainId": 137,
"toChainSlug": "polygon",
"toChainTxHash": "0x0554846ce55a79b5b635230263a7252c6b7749d1c32a845185e5f35d1aeb150b",
"updatedAt": "2024-04-03T12:36:46.810Z",
"createdAt": "2024-04-03T12:34:32.846Z",
"id": 238617,
..................
}
Polling Transaction Status
For the sake of user experience, while a live transaction is processing, it’s best to periodically poll the transaction status endpoint to check if the transaction is complete. This will keep your users informed about the status of their transactions in real time.
Let's define a function for checking a transaction's status:
async function getStatus({ id, txHash, projectId }) {
const result = await axios.get(
'https://swap.prod.swing.xyz/v0/transfer/status',
{
params: {
id,
txHash,
projectId,
},
},
);
return result.data;
}
Polling the transaction status endpoint means that we will be periodically querying the /status
endpoint until the status property in the response is no longer one of the following:
- Submitted
- Not Sent
- Pending Source Chain
- Pending Destination Chain
Once the transaction status does not match any of the following, it indicates that the /send
endpoint has returned a status message specifying whether the transaction has failed or completed.
Next, We will use setTimeout()
to execute our getStatus()
function every 5000 milliseconds recursively until the conditions stated above are met.
const pendingStatuses = [
'Submitted',
'Not Sent',
'Pending Source Chain',
'Pending Destination Chain',
];
const transactionPollingDuration = 5000;
async function pollTransactionStatus({ transId, txHash, projectId }) {
const transactionStatus = await getTransStatus({
transId,
txHash,
projectId,
});
if (pendingStatuses.includes(transactionStatus?.status)) {
setTimeout(
() => pollTransactionStatus({ transId, txHash, projectId }),
transactionPollingDuration,
);
} else {
console.log(
transactionStatus === 'Completed'
? 'Transaction Completed'
: 'Transaction Failed',
);
}
}
Next, we will call our pollTransactionStatus()
function right after executing our callData
:
const transaction = await sendTransaction();
let txData = {
data: transaction.tx.data,
from: transaction.tx.from,
to: transaction.tx.to,
value: transaction.tx.value,
gasLimit: transaction.tx.gas,
};
const txHash = await executeTxData(txData);
await pollTransactionStatus({
id: transaction.id,
txHash,
projectId: 'replug',
});