Choosing an Integration
If it's your first time building a cross-chain application, you might be unsure of which integration type would be the best fit for you and your team. The aim of this guide is to help you decide which of Swing's cross-chain integrations will best fit your needs.
Before moving on, it's important to note that all our integration options have full cross-chain capabilities and essentially function the same way. So, regardless of your integration choice, the core results will be the same.
Quick Comparison Overview
Below is a quick comparison of our integration options to give you an idea of what to expect as we go through this guide.
Operation | SDK | API | Widget |
---|---|---|---|
EVM {<>} Solana Cross Chain Swaps | ✅ | ✅ | ✅ |
EVM {<>} Bitcoin Cross Chain Swaps | ❌ | ✅ | ❌ |
EVM {<>} EVM Cross Chain Swaps | ✅ | ✅ | ✅ |
EVM {<>} EVM Single Chain Swaps | ✅ | ✅ | ✅ |
EVM {<>} EVM Cross Chain Staking/Deposits | ✅ | ✅ | ✅ |
EVM {<>} EVM Single chain Staking/Deposits | ✅ | ✅ | ✅ |
EVM {<>} IBC Cross Chain Swaps | ✅ | ✅ | ✅ |
IBC {<>} IBC Cross Chain Swaps | ✅ | ✅ | ✅ |
Swing supports both cross-chain and single-chain swaps, as well as cross-chain and single-chain staking/deposits. Check supported chains, bridges and liquidity.
Why choose Swing's Widget?
Our widget is a pre-built, fully customizable, embeddable UI component, that can be integrated into your dApp with just a few lines of code.
import { Swap } from '@swing.xyz/ui';
// Import the theme CSS directly within your component or within another css file
import '@swing.xyz/ui/theme.css';
function Component() {
return <Swap projectId="swing-project-id" />;
}
Our Widget is built primarily as an embeddable component for React, but we also offer support to other web frameworks via our HTML Widget.
If you're an Angular developer, you can read this guide on how to setup our Widget in Angular.
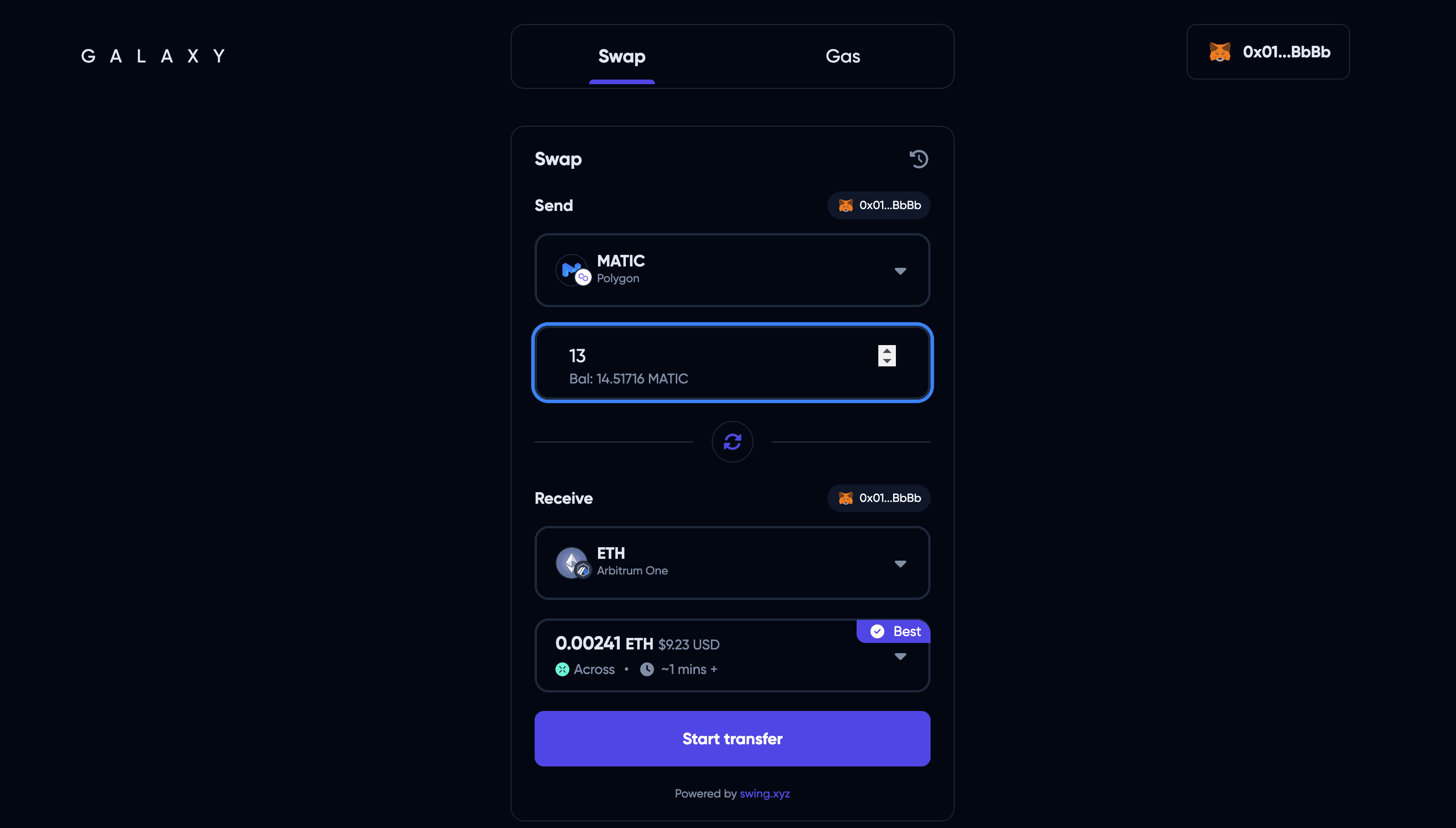
You can try out our widget here.
We recommend using our Widget if:
- You want to save time: If you're looking to quickly go to market and don't mind using a pre-built UI, our Widget is your best bet!
Pros and Cons of using Swing's Widget
Though our widget is a perfect solution for developers looking to go to market quickly, there are some caviats, let's discuss them:
Pros:
- Requires minimal to no effort to integrate, enabling developers to achieve same day delivery with full cross-chain capabilities.
- Works with and can be integrated into any
web application
. Simply import and embed the widget into your web app.
Cons:
- Although you can customize the
CSS
andtitle
of the Widget, you're restricted from customizing the Widget's components and layouts.
Widget Examples/Starter Projects
You can check out our examples and starter projects with our Widget integrated, which you can use as references to build your apps here.
Here are a few example customers using the Widget
For reference, here is a list of a few customers of ours who have integrated our Widget. Check if your needs align with theirs:
Customer | Type | Description |
---|---|---|
Orbiter One | DeFi | Cross-chain liquidity lending services |
Attack Wagon | GameFi | A web3 decentralized gaming finance |
Locus Finance | Staking Protocol | Decentralized asset management made simple. |
Galaxy | DEX | Bridge & swap across multiple chains |
If our Widget integration is the right choice for you, check out our Widget Guide.
Why choose Swing's SDK?
Our SDK is an easy-to-use JavaScript
/TypeScript
implementation of Swing's API that enables developers to quickly integrate Swing's services.
Our SDK is most suitable for developers building with JavaScript and TypeScript stacks and requires a minimum of Node v18
or later.
const swingSDK = new SwingSDK({
projectId: 'replug',
debug: true,
});
await swingSdk.wallet.connect(window.ethereum);
//Define transfer params (ETH <> SOL)
const params = {
amount: '1',
fromChain: 'ethereum',
fromToken: 'ETH',
toChain: 'solana',
toToken: 'SOL',
fromUserAddress: 'ethereum-user-address',
toUserAddress: 'solana-user-address',
};
//Get a transaction quote and route
const quote = await swingSdk.getQuote(params);
//Listen for transaction events from Swing
swingSdk.on('TRANSFER', async (transferStepStatus, transferResults) => {
console.log('TRANSFER:', transferStepStatus, transferResults);
});
//Begin a transaction
await swingSdk.transfer(quote.routes[0], params);
We recommend using our SDK if:
- You're building primarily with either
JavaScript
orTypeScript
. Our SDK is built with JavaScript/TypeScript and works with all JavaScript and TypeScript frameworks. - You want to keep a clean workflow: You can do more with less coding as our SDK handles all API fetch operations.
Pros and Cons of using Swing's SDK
Integrating our SDK has some key advantages over integrating our APIs. Let's delve into them.
Pros:
- Our SDK supports all popular wallet connector such as
MetaMask
,Phantom
,Rabby
, etc, allowing direct interaction with a user's wallet and eliminating the need for developers to write wallet-specific code into their dApps. You can learn more about our supported wallets here. - Our SDK handles all API fetch requests, eliminating the need for developers to write fetch requests from scratch. This in-turn, boosts development time.
- Our SDK is strongly typed and exports helper
types
for developers using TypeScript, eliminating the need for developers to create their own types from scratch. - Our SDK includes events that provide real-time feedback on actions triggered from inside a user's wallet and real-time data from ongoing transactions.
Cons:
- Our SDK supports only
JavaScript
andTypeScript
.
Need support for another language? No problem! Just give our friendly support team a shout.
For JavaScript developers considering integrating our APIs instead of our SDK, it's important to note that our SDK exports two strongly typed objects: platformAPI
and crossChainAPI
. These objects act as wrappers around our APIs and include all the response objects available in our APIs.
This is great news as you can get the best of both worlds by essentially combining our APIs and our SDK together.
const chains = swingSDK.chains;
//Get Chains using `platformAPI`
const chains = await swingSDK.platformAPI.GET('/chains', {
params: {
query: {
type: 'evm',
},
},
});
SDK Examples/Starter Projects
You can check out our examples and starter projects with our SDK integrated, which you can use as references to build your apps here.
Here are a few example customers using the SDK
For reference, here is a list of our customers who have integrated our SDK. Check if your needs align with theirs:
Customer | Type | Description |
---|---|---|
Rampanalysis | CEX | Intelligent Fiat On/Off Ramp |
Staking Rewards | Aggregator | The Staking Explorer |
If our SDK integration is the right choice for you, check out our SDK Guide.
Why choose Swing's API?
Swing's APIs form the foundation of all our cross-chain tools. Both our SDK and Widget are built using these APIs and operate them behind the scenes.
Our APIs are most suitable for developers who aren't working with a JavaScript
/TypeScript
stack. Meaning developers building primary with any other language like C# or Python will have to resort to using our APIs.
If you're building with JavaScript/TypeScript, we recommend checking out our SDK Guides or Widget Guides
We recommend using our APIs if:
-
You're building with any programming language other than JavaScript or using a Non-JavaScript framework or library: If your development environment involves languages such as
Python
,Ruby
,Java
, or any other programming language besidesJavaScript
, or if you're using frameworks or libraries that are not based on JavaScript, then feel free to use our APIs. -
Your app is running on a legacy version of Node.js: All of our
JavaScript
/TypeScript
tools run on Node v18 or later. If your dApp is using an older version of Node.js that might not be supported by our SDK, you can integrate our APIs without having to update your Node.js version.
Pros and Cons of using Swing's APIs
Although our APIs are specificly targetted towards non JavaScript
/TypeScript
developers, there are some advantages to using our APIs.
Pros:
- You can integrate our services using any programming language, stack, or framework, including on mobile.
Cons:
- Takes longer to integrate when compared to our SDK as developers have to build all their
Dtos
/types
/interfaces
and API fetch requests from scratch.
API Examples/Starter Projects
You can check out our examples and starter projects with our API integrated, which you can use as references to build your apps here.
Here are a few example customers using the API
For reference, here is a list of our customers who have integrated our APIs. Check if your needs align with theirs:
Customer | Type | Description |
---|---|---|
Chainspot | Aggregator | Bridge and swap your assets |
de.Farm | DEX | SocialFi for Onchain Degens |
Xenify | DEX | Cross-chain meta-aggregator of aggregators |
If our API integration is the right choice for you, check out our API Guide.
Conclusion
We hope this guide has helped you decide which of our integration options works best for you. We can't wait to see what you're building!
SDK Guides
Build your own any-to-any cross-chain UI and interact with Swing API & web3 wallets.
🎯 A great choice for JavaScript developers looking to build quickly
Widget Guides
Learn how to embed the cross-chain widget into your app with a few lines of code.
🎯 A slick pre-built plug and play UI for developers looking to quickly go to market.
API Guides
Learn the key API principles and how to integrate endpoints into dApps, web or mobile apps.
🎯 Perfect for developers who aren't using a JavaScript stack!
If you're still unsure which integration type is perfect for you or your team, check out our support channels to reach out to us, and we'll get back to you as soon as possible.
Happy building!